I love facebook API it's neat, so I had developed Facebook like Autosuggestion user search with jQuery, Ajax and PHP. It's simple and clean just you have to change the database details.
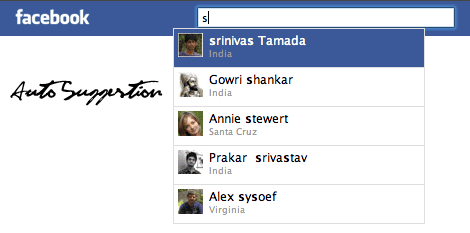
Take a look at live demo, search word " sri "


Download the Script. Edit Config.php change the database details.
Database
create database table with name "test_user_data"
CREATE TABLE test_user_data
(
uid INT AUTO_INCREMENT PRIMARY KEY,
fname VARCHAR(25),
lname VARCHAR(25),
country VARCHAR(25),
img VARCHAR(50)
);
(
uid INT AUTO_INCREMENT PRIMARY KEY,
fname VARCHAR(25),
lname VARCHAR(25),
country VARCHAR(25),
img VARCHAR(50)
);
Auto.html
contains jquery(javascript) and HTML Code. Take a look at input field class values search
<script type="text/javascript" src="jquery.js"></script>
</script>
$(document).ready(function(){
$(".search").keyup(function()
{
var searchbox = $(this).val();
var dataString = 'searchword='+ searchbox;
if(searchbox=='')
{}
else
{
$.ajax({
type: "POST",
url: "search.php",
data: dataString,
cache: false,
success: function(html)
{
$("#display").html(html).show();
}
});
}return false;
});
});
</script>
<div id="display">
</script>
$(document).ready(function(){
$(".search").keyup(function()
{
var searchbox = $(this).val();
var dataString = 'searchword='+ searchbox;
if(searchbox=='')
{}
else
{
$.ajax({
type: "POST",
url: "search.php",
data: dataString,
cache: false,
success: function(html)
{
$("#display").html(html).show();
}
});
}return false;
});
});
</script>
<input type="text"color: blue;">search" id="searchbox" />
<div id="display">
</div>
search.php
Contains PHP code. Display search results
<?php
include('config.php');
if($_POST)
{
$q=$_POST['searchword'];
$sql_res=
mysql_query("select * from test_user_data where fname like '%$q%' or lname like '%$q%' order by uid LIMIT 5");
while($row=mysql_fetch_array($sql_res))
{
$fname=$row['fname'];
$lname=$row['lname'];
$img=$row['img'];
$country=$row['country'];
$re_fname='<b>'.$q.'</b>';
$re_lname='<b>'.$q.'</b>';
$final_fname = str_ireplace($q, $re_fname, $fname);
$final_lname = str_ireplace($q, $re_lname, $lname);
?>
<divcolor: blue;">display_box" align="left">
<img src="user_img/
<?php echo $img; ?>" />
<?php echo $final_fname; ?>
<?php echo $final_lname; ?><br/>
<?php echo $country; ?>
</div>
<?php
}
}
else
{}
?>
Using Watermark Input plugin
To show information about the contents of a text field.
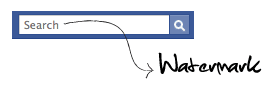
<script type="text/javascript" src="jquery.watermarkinput.js"></script>
</script>
jQuery(function($){
$("#searchbox").Watermark("Search");
});
</script>
</script>
jQuery(function($){
$("#searchbox").Watermark("Search");
});
</script>
CSS
id #diplay overflow : hidden
*{margin:0px}
{
width:250px;
border:solid 1px #000;
padding:3px;
}
{
width:250px;
display:none;
float:right; margin-right:30px;
border-left:solid 1px #dedede;
border-right:solid 1px #dedede;
border-bottom:solid 1px #dedede;
overflow:hidden;
}
{
padding:4px;
border-top:solid 1px #dedede;
font-size:12px;
height:30px;
}
{
background:#3b5998;
color:#FFFFFF;
}
#searchbox
{
width:250px;
border:solid 1px #000;
padding:3px;
}
#display
{
width:250px;
display:none;
float:right; margin-right:30px;
border-left:solid 1px #dedede;
border-right:solid 1px #dedede;
border-bottom:solid 1px #dedede;
overflow:hidden;
}
.display_box
{
padding:4px;
border-top:solid 1px #dedede;
font-size:12px;
height:30px;
}
.display_box:hover
{
background:#3b5998;
color:#FFFFFF;
}
if any queries just post a comment.